mirror of
https://github.com/yusing/godoxy.git
synced 2025-05-20 20:52:33 +02:00
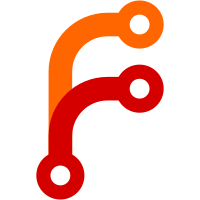
- Incorrect name being shown on dashboard "Proxies page" - Apps being shown when homepage.show is false - Load balanced routes are shown on homepage instead of the load balancer - Route with idlewatcher will now be removed on container destroy - Idlewatcher panic - Performance improvement - Idlewatcher infinitely loading - Reload stucked / not working properly - Streams stuck on shutdown / reload - etc... Added: - support idlewatcher for loadbalanced routes - partial implementation for stream type idlewatcher Issues: - graceful shutdown
57 lines
1.4 KiB
Go
57 lines
1.4 KiB
Go
package docker
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"time"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/container"
|
|
"github.com/docker/docker/client"
|
|
E "github.com/yusing/go-proxy/internal/error"
|
|
)
|
|
|
|
type ClientInfo struct {
|
|
Client Client
|
|
Containers []types.Container
|
|
}
|
|
|
|
var listOptions = container.ListOptions{
|
|
// created|restarting|running|removing|paused|exited|dead
|
|
// Filters: filters.NewArgs(
|
|
// filters.Arg("status", "created"),
|
|
// filters.Arg("status", "restarting"),
|
|
// filters.Arg("status", "running"),
|
|
// filters.Arg("status", "paused"),
|
|
// filters.Arg("status", "exited"),
|
|
// ),
|
|
All: true,
|
|
}
|
|
|
|
func GetClientInfo(clientHost string, getContainer bool) (*ClientInfo, E.NestedError) {
|
|
dockerClient, err := ConnectClient(clientHost)
|
|
if err.HasError() {
|
|
return nil, E.FailWith("connect to docker", err)
|
|
}
|
|
defer dockerClient.Close()
|
|
|
|
ctx, cancel := context.WithTimeoutCause(context.Background(), 3*time.Second, errors.New("docker client connection timeout"))
|
|
defer cancel()
|
|
|
|
var containers []types.Container
|
|
if getContainer {
|
|
containers, err = E.Check(dockerClient.ContainerList(ctx, listOptions))
|
|
if err.HasError() {
|
|
return nil, E.FailWith("list containers", err)
|
|
}
|
|
}
|
|
|
|
return &ClientInfo{
|
|
Client: dockerClient,
|
|
Containers: containers,
|
|
}, nil
|
|
}
|
|
|
|
func IsErrConnectionFailed(err error) bool {
|
|
return client.IsErrConnectionFailed(err)
|
|
}
|