mirror of
https://github.com/yusing/godoxy.git
synced 2025-05-20 04:42:33 +02:00
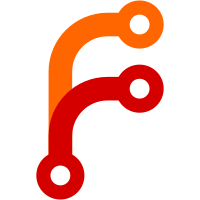
* fix: improved sync.Pool handling
* refactor: ping if-flow and remove timeout
* refactor: enhance favicon fetching with context support and improve cache management
- Added context support to favicon fetching functions to handle timeouts and cancellations.
- Improved cache entry structure to include content type and utilize atomic values for last access time.
- Implemented maximum cache size and entry limits to optimize memory usage.
- Updated error handling for HTTP requests and refined the logic for managing redirects.
* fix: log formatting
* feat(pool): add checkExists method to debug build to detect unexpected behavior
* chore: cont. 0866feb
* refactor: unify route handling by consolidating route query methods with Pool
- Replaced direct calls to routequery with a new routes package for better organization and maintainability.
- Updated various components to utilize the new routes methods for fetching health information, homepage configurations, and route aliases.
- Enhanced the overall structure of the routing logic to improve clarity and reduce redundancy.
* chore: uncomment icon list cache code
* refactor: update task management code
- Rename needFinish to waitFinish
- Fixed some tasks not being waited they should be
- Adjusted mutex usage in the directory watcher to utilize read-write locks for improved concurrency management.
* refactor: enhance idlewatcher logging and exit handling
* fix(server): ensure HTTP handler is set only if initialized
* refactor(accesslog): replace JSON log entry struct with zerolog for improved logging efficiency, updated test
* refactor: remove test run code
---------
Co-authored-by: yusing <yusing@6uo.me>
89 lines
1.9 KiB
Go
89 lines
1.9 KiB
Go
package task
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"os"
|
|
"os/signal"
|
|
"syscall"
|
|
"time"
|
|
|
|
"github.com/yusing/go-proxy/internal/logging"
|
|
F "github.com/yusing/go-proxy/internal/utils/functional"
|
|
)
|
|
|
|
var ErrProgramExiting = errors.New("program exiting")
|
|
|
|
var (
|
|
root = newRoot()
|
|
allTasks = F.NewSet[*Task]()
|
|
)
|
|
|
|
func testCleanup() {
|
|
root = newRoot()
|
|
allTasks.Clear()
|
|
}
|
|
|
|
// RootTask returns a new Task with the given name, derived from the root context.
|
|
func RootTask(name string, needFinish bool) *Task {
|
|
return root.Subtask(name, needFinish)
|
|
}
|
|
|
|
func newRoot() *Task {
|
|
t := &Task{
|
|
name: "root",
|
|
childrenDone: make(chan struct{}),
|
|
finished: make(chan struct{}),
|
|
}
|
|
t.ctx, t.cancel = context.WithCancelCause(context.Background())
|
|
return t
|
|
}
|
|
|
|
func RootContext() context.Context {
|
|
return root.ctx
|
|
}
|
|
|
|
func RootContextCanceled() <-chan struct{} {
|
|
return root.ctx.Done()
|
|
}
|
|
|
|
func OnProgramExit(about string, fn func()) {
|
|
root.OnFinished(about, fn)
|
|
}
|
|
|
|
// GracefulShutdown waits for all tasks to finish, up to the given timeout.
|
|
//
|
|
// If the timeout is exceeded, it prints a list of all tasks that were
|
|
// still running when the timeout was reached, and their current tree
|
|
// of subtasks.
|
|
func GracefulShutdown(timeout time.Duration) (err error) {
|
|
go root.Finish(ErrProgramExiting)
|
|
|
|
after := time.After(timeout)
|
|
for {
|
|
select {
|
|
case <-root.finished:
|
|
return
|
|
case <-after:
|
|
logging.Warn().Msgf("Timeout waiting for %d tasks to finish", allTasks.Size())
|
|
for t := range allTasks.Range {
|
|
logging.Warn().Msgf("Task %s is still running", t.name)
|
|
}
|
|
return context.DeadlineExceeded
|
|
}
|
|
}
|
|
}
|
|
|
|
func WaitExit(shutdownTimeout int) {
|
|
sig := make(chan os.Signal, 1)
|
|
signal.Notify(sig, syscall.SIGINT)
|
|
signal.Notify(sig, syscall.SIGTERM)
|
|
signal.Notify(sig, syscall.SIGHUP)
|
|
|
|
// wait for signal
|
|
<-sig
|
|
|
|
// gracefully shutdown
|
|
logging.Info().Msg("shutting down")
|
|
_ = GracefulShutdown(time.Second * time.Duration(shutdownTimeout))
|
|
}
|