mirror of
https://github.com/yusing/godoxy.git
synced 2025-05-20 04:42:33 +02:00
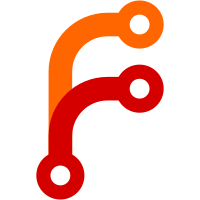
* fix: improved sync.Pool handling
* refactor: ping if-flow and remove timeout
* refactor: enhance favicon fetching with context support and improve cache management
- Added context support to favicon fetching functions to handle timeouts and cancellations.
- Improved cache entry structure to include content type and utilize atomic values for last access time.
- Implemented maximum cache size and entry limits to optimize memory usage.
- Updated error handling for HTTP requests and refined the logic for managing redirects.
* fix: log formatting
* feat(pool): add checkExists method to debug build to detect unexpected behavior
* chore: cont. 0866feb
* refactor: unify route handling by consolidating route query methods with Pool
- Replaced direct calls to routequery with a new routes package for better organization and maintainability.
- Updated various components to utilize the new routes methods for fetching health information, homepage configurations, and route aliases.
- Enhanced the overall structure of the routing logic to improve clarity and reduce redundancy.
* chore: uncomment icon list cache code
* refactor: update task management code
- Rename needFinish to waitFinish
- Fixed some tasks not being waited they should be
- Adjusted mutex usage in the directory watcher to utilize read-write locks for improved concurrency management.
* refactor: enhance idlewatcher logging and exit handling
* fix(server): ensure HTTP handler is set only if initialized
* refactor(accesslog): replace JSON log entry struct with zerolog for improved logging efficiency, updated test
* refactor: remove test run code
---------
Co-authored-by: yusing <yusing@6uo.me>
56 lines
1.5 KiB
Go
56 lines
1.5 KiB
Go
package json
|
|
|
|
import (
|
|
"reflect"
|
|
|
|
"github.com/bytedance/sonic"
|
|
"github.com/yusing/go-proxy/internal/utils/synk"
|
|
)
|
|
|
|
type Marshaler interface {
|
|
MarshalJSONTo(buf []byte) []byte
|
|
}
|
|
|
|
var (
|
|
Unmarshal = sonic.Unmarshal
|
|
Valid = sonic.Valid
|
|
NewDecoder = sonic.ConfigDefault.NewDecoder
|
|
)
|
|
|
|
// Marshal returns the JSON encoding of v.
|
|
//
|
|
// It's like json.Marshal, but with some differences:
|
|
//
|
|
// - It supports custom Marshaler interface (MarshalJSONTo(buf []byte) []byte)
|
|
// to allow further optimizations.
|
|
//
|
|
// - It leverages the strutils library.
|
|
//
|
|
// - It drops the need to implement Marshaler or json.Marshaler by supports extra field tags:
|
|
//
|
|
// `byte_size` to format the field to human readable size.
|
|
//
|
|
// `unix_time` to format the uint64 field to string date-time without specifying MarshalJSONTo.
|
|
//
|
|
// `use_marshaler` to force using the custom marshaler for primitive types declaration (e.g. `type Status int`).
|
|
//
|
|
// - It corrects the behavior of *url.URL and time.Duration.
|
|
//
|
|
// - It does not support maps other than string-keyed maps.
|
|
func Marshal(v any) ([]byte, error) {
|
|
buf := bytesPool.Get()
|
|
defer bytesPool.Put(buf)
|
|
return cloneBytes(appendMarshal(reflect.ValueOf(v), buf)), nil
|
|
}
|
|
|
|
func MarshalTo(v any, buf []byte) []byte {
|
|
return appendMarshal(reflect.ValueOf(v), buf)
|
|
}
|
|
|
|
const initBufSize = 4096
|
|
|
|
var bytesPool = synk.NewBytesPool(initBufSize, synk.DefaultMaxBytes)
|
|
|
|
func cloneBytes(buf []byte) (res []byte) {
|
|
return append(res, buf...)
|
|
}
|