mirror of
https://github.com/yusing/godoxy.git
synced 2025-07-13 01:14:04 +02:00
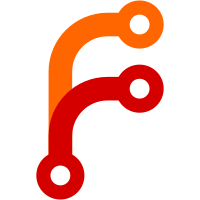
- Incorrect name being shown on dashboard "Proxies page" - Apps being shown when homepage.show is false - Load balanced routes are shown on homepage instead of the load balancer - Route with idlewatcher will now be removed on container destroy - Idlewatcher panic - Performance improvement - Idlewatcher infinitely loading - Reload stucked / not working properly - Streams stuck on shutdown / reload - etc... Added: - support idlewatcher for loadbalanced routes - partial implementation for stream type idlewatcher Issues: - graceful shutdown
37 lines
928 B
Go
37 lines
928 B
Go
package idlewatcher
|
|
|
|
import (
|
|
"bytes"
|
|
_ "embed"
|
|
"fmt"
|
|
"strings"
|
|
"text/template"
|
|
)
|
|
|
|
type templateData struct {
|
|
CheckRedirectHeader string
|
|
Title string
|
|
Message string
|
|
}
|
|
|
|
//go:embed html/loading_page.html
|
|
var loadingPage []byte
|
|
var loadingPageTmpl = template.Must(template.New("loading_page").Parse(string(loadingPage)))
|
|
|
|
const headerCheckRedirect = "X-Goproxy-Check-Redirect"
|
|
|
|
func (w *Watcher) makeLoadingPageBody() []byte {
|
|
msg := fmt.Sprintf("%s is starting...", w.ContainerName)
|
|
|
|
data := new(templateData)
|
|
data.CheckRedirectHeader = headerCheckRedirect
|
|
data.Title = w.ContainerName
|
|
data.Message = strings.ReplaceAll(msg, " ", " ")
|
|
|
|
buf := bytes.NewBuffer(make([]byte, len(loadingPage)+len(data.Title)+len(data.Message)+len(headerCheckRedirect)))
|
|
err := loadingPageTmpl.Execute(buf, data)
|
|
if err != nil { // should never happen in production
|
|
panic(err)
|
|
}
|
|
return buf.Bytes()
|
|
}
|