mirror of
https://github.com/yusing/godoxy.git
synced 2025-07-15 18:04:02 +02:00
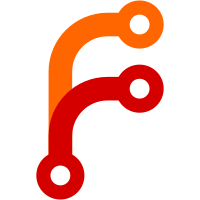
- Incorrect name being shown on dashboard "Proxies page" - Apps being shown when homepage.show is false - Load balanced routes are shown on homepage instead of the load balancer - Route with idlewatcher will now be removed on container destroy - Idlewatcher panic - Performance improvement - Idlewatcher infinitely loading - Reload stucked / not working properly - Streams stuck on shutdown / reload - etc... Added: - support idlewatcher for loadbalanced routes - partial implementation for stream type idlewatcher Issues: - graceful shutdown
113 lines
2 KiB
Go
113 lines
2 KiB
Go
package utils
|
|
|
|
import (
|
|
"errors"
|
|
"os"
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/yusing/go-proxy/internal/common"
|
|
E "github.com/yusing/go-proxy/internal/error"
|
|
)
|
|
|
|
func init() {
|
|
if common.IsTest {
|
|
os.Args = append([]string{os.Args[0], "-test.v"}, os.Args[1:]...)
|
|
}
|
|
}
|
|
|
|
func IgnoreError[Result any](r Result, _ error) Result {
|
|
return r
|
|
}
|
|
|
|
func ExpectNoError(t *testing.T, err error) {
|
|
t.Helper()
|
|
if err != nil && !reflect.ValueOf(err).IsNil() {
|
|
t.Errorf("expected err=nil, got %s", err)
|
|
t.FailNow()
|
|
}
|
|
}
|
|
|
|
func ExpectError(t *testing.T, expected error, err error) {
|
|
t.Helper()
|
|
if !errors.Is(err, expected) {
|
|
t.Errorf("expected err %s, got %s", expected, err)
|
|
t.FailNow()
|
|
}
|
|
}
|
|
|
|
func ExpectError2(t *testing.T, input any, expected error, err error) {
|
|
t.Helper()
|
|
if !errors.Is(err, expected) {
|
|
t.Errorf("%v: expected err %s, got %s", input, expected, err)
|
|
t.FailNow()
|
|
}
|
|
}
|
|
|
|
func ExpectEqual[T comparable](t *testing.T, got T, want T) {
|
|
t.Helper()
|
|
if got != want {
|
|
t.Errorf("expected:\n%v, got\n%v", want, got)
|
|
t.FailNow()
|
|
}
|
|
}
|
|
|
|
func ExpectEqualAny[T comparable](t *testing.T, got T, wants []T) {
|
|
t.Helper()
|
|
for _, want := range wants {
|
|
if got == want {
|
|
return
|
|
}
|
|
}
|
|
t.Errorf("expected any of:\n%v, got\n%v", wants, got)
|
|
t.FailNow()
|
|
}
|
|
|
|
func ExpectDeepEqual[T any](t *testing.T, got T, want T) {
|
|
t.Helper()
|
|
if !reflect.DeepEqual(got, want) {
|
|
t.Errorf("expected:\n%v, got\n%v", want, got)
|
|
t.FailNow()
|
|
}
|
|
}
|
|
|
|
func ExpectTrue(t *testing.T, got bool) {
|
|
t.Helper()
|
|
if !got {
|
|
t.Error("expected true")
|
|
t.FailNow()
|
|
}
|
|
}
|
|
|
|
func ExpectFalse(t *testing.T, got bool) {
|
|
t.Helper()
|
|
if got {
|
|
t.Error("expected false")
|
|
t.FailNow()
|
|
}
|
|
}
|
|
|
|
func ExpectType[T any](t *testing.T, got any) (_ T) {
|
|
t.Helper()
|
|
tExpect := reflect.TypeFor[T]()
|
|
_, ok := got.(T)
|
|
if !ok {
|
|
t.Fatalf("expected type %s, got %s", tExpect, reflect.TypeOf(got).Elem())
|
|
return
|
|
}
|
|
return got.(T)
|
|
}
|
|
|
|
func Must[T any](v T, err E.NestedError) T {
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return v
|
|
}
|
|
|
|
func Must2[T any](v T, err error) T {
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return v
|
|
}
|