mirror of
https://github.com/yusing/godoxy.git
synced 2025-07-05 22:24:02 +02:00
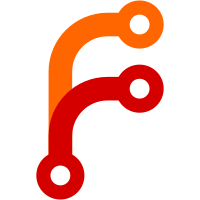
* feat: idle sleep for proxmox LXCs * refactor: replace deprecated docker api types * chore(api): remove debug task list endpoint * refactor: move servemux to gphttp/servemux; favicon.go to v1/favicon * refactor: introduce Pool interface, move agent_pool to agent module * refactor: simplify api code * feat: introduce debug api * refactor: remove net.URL and net.CIDR types, improved unmarshal handling * chore: update Makefile for debug build tag, update README * chore: add gperr.Unwrap method * feat: relative time and duration formatting * chore: add ROOT_DIR environment variable, refactor * migration: move homepage override and icon cache to $BASE_DIR/data, add migration code * fix: nil dereference on marshalling service health * fix: wait for route deletion * chore: enhance tasks debuggability * feat: stdout access logger and MultiWriter * fix(agent): remove agent properly on verify error * fix(metrics): disk exclusion logic and added corresponding tests * chore: update schema and prettify, fix package.json and Makefile * fix: I/O buffer not being shrunk before putting back to pool * feat: enhanced error handling module * chore: deps upgrade * feat: better value formatting and handling --------- Co-authored-by: yusing <yusing@6uo.me>
69 lines
1.6 KiB
Go
69 lines
1.6 KiB
Go
package accesslog
|
|
|
|
import (
|
|
"errors"
|
|
|
|
"github.com/yusing/go-proxy/internal/utils"
|
|
)
|
|
|
|
type (
|
|
Format string
|
|
Filters struct {
|
|
StatusCodes LogFilter[*StatusCodeRange] `json:"status_codes"`
|
|
Method LogFilter[HTTPMethod] `json:"method"`
|
|
Host LogFilter[Host] `json:"host"`
|
|
Headers LogFilter[*HTTPHeader] `json:"headers"` // header exists or header == value
|
|
CIDR LogFilter[*CIDR] `json:"cidr"`
|
|
}
|
|
Fields struct {
|
|
Headers FieldConfig `json:"headers"`
|
|
Query FieldConfig `json:"query"`
|
|
Cookies FieldConfig `json:"cookies"`
|
|
}
|
|
Config struct {
|
|
BufferSize int `json:"buffer_size"`
|
|
Format Format `json:"format" validate:"oneof=common combined json"`
|
|
Path string `json:"path"`
|
|
Stdout bool `json:"stdout"`
|
|
Filters Filters `json:"filters"`
|
|
Fields Fields `json:"fields"`
|
|
Retention *Retention `json:"retention"`
|
|
}
|
|
)
|
|
|
|
var (
|
|
FormatCommon Format = "common"
|
|
FormatCombined Format = "combined"
|
|
FormatJSON Format = "json"
|
|
)
|
|
|
|
const DefaultBufferSize = 64 * 1024 // 64KB
|
|
|
|
func (cfg *Config) Validate() error {
|
|
if cfg.Path == "" && !cfg.Stdout {
|
|
return errors.New("path or stdout is required")
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func DefaultConfig() *Config {
|
|
return &Config{
|
|
BufferSize: DefaultBufferSize,
|
|
Format: FormatCombined,
|
|
Fields: Fields{
|
|
Headers: FieldConfig{
|
|
Default: FieldModeDrop,
|
|
},
|
|
Query: FieldConfig{
|
|
Default: FieldModeKeep,
|
|
},
|
|
Cookies: FieldConfig{
|
|
Default: FieldModeDrop,
|
|
},
|
|
},
|
|
}
|
|
}
|
|
|
|
func init() {
|
|
utils.RegisterDefaultValueFactory(DefaultConfig)
|
|
}
|