mirror of
https://github.com/yusing/godoxy.git
synced 2025-07-18 18:54:04 +02:00
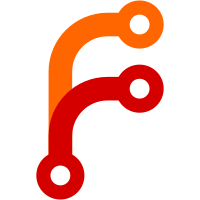
* chore: replace gopkg.in/yaml.v3 vs goccy/go-yaml; replace encoding/json with bytedance/sonic * fix: yaml unmarshal panic * feat: custom json marshaler implementation * chore: fix import and err marshal handling --------- Co-authored-by: yusing <yusing@6uo.me>
42 lines
1.1 KiB
Go
42 lines
1.1 KiB
Go
package certapi
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/yusing/go-proxy/pkg/json"
|
|
|
|
config "github.com/yusing/go-proxy/internal/config/types"
|
|
)
|
|
|
|
type CertInfo struct {
|
|
Subject string `json:"subject"`
|
|
Issuer string `json:"issuer"`
|
|
NotBefore int64 `json:"not_before"`
|
|
NotAfter int64 `json:"not_after"`
|
|
DNSNames []string `json:"dns_names"`
|
|
EmailAddresses []string `json:"email_addresses"`
|
|
}
|
|
|
|
func GetCertInfo(w http.ResponseWriter, r *http.Request) {
|
|
autocert := config.GetInstance().AutoCertProvider()
|
|
if autocert == nil {
|
|
http.Error(w, "autocert is not enabled", http.StatusNotFound)
|
|
return
|
|
}
|
|
|
|
cert, err := autocert.GetCert(nil)
|
|
if err != nil {
|
|
http.Error(w, err.Error(), http.StatusInternalServerError)
|
|
return
|
|
}
|
|
|
|
certInfo := CertInfo{
|
|
Subject: cert.Leaf.Subject.CommonName,
|
|
Issuer: cert.Leaf.Issuer.CommonName,
|
|
NotBefore: cert.Leaf.NotBefore.Unix(),
|
|
NotAfter: cert.Leaf.NotAfter.Unix(),
|
|
DNSNames: cert.Leaf.DNSNames,
|
|
EmailAddresses: cert.Leaf.EmailAddresses,
|
|
}
|
|
json.NewEncoder(w).Encode(&certInfo)
|
|
}
|