mirror of
https://github.com/yusing/godoxy.git
synced 2025-05-21 21:12:34 +02:00
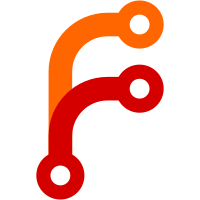
* feat: idle sleep for proxmox LXCs * refactor: replace deprecated docker api types * chore(api): remove debug task list endpoint * refactor: move servemux to gphttp/servemux; favicon.go to v1/favicon * refactor: introduce Pool interface, move agent_pool to agent module * refactor: simplify api code * feat: introduce debug api * refactor: remove net.URL and net.CIDR types, improved unmarshal handling * chore: update Makefile for debug build tag, update README * chore: add gperr.Unwrap method * feat: relative time and duration formatting * chore: add ROOT_DIR environment variable, refactor * migration: move homepage override and icon cache to $BASE_DIR/data, add migration code * fix: nil dereference on marshalling service health * fix: wait for route deletion * chore: enhance tasks debuggability * feat: stdout access logger and MultiWriter * fix(agent): remove agent properly on verify error * fix(metrics): disk exclusion logic and added corresponding tests * chore: update schema and prettify, fix package.json and Makefile * fix: I/O buffer not being shrunk before putting back to pool * feat: enhanced error handling module * chore: deps upgrade * feat: better value formatting and handling --------- Co-authored-by: yusing <yusing@6uo.me>
86 lines
2.3 KiB
Go
86 lines
2.3 KiB
Go
package accesslog_test
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
"time"
|
|
|
|
. "github.com/yusing/go-proxy/internal/net/gphttp/accesslog"
|
|
"github.com/yusing/go-proxy/internal/task"
|
|
"github.com/yusing/go-proxy/internal/utils/strutils"
|
|
. "github.com/yusing/go-proxy/internal/utils/testing"
|
|
)
|
|
|
|
func TestParseLogTime(t *testing.T) {
|
|
tests := []string{
|
|
`{"foo":"bar","time":"%s","bar":"baz"}`,
|
|
`example.com 192.168.1.1 - - [%s] "GET / HTTP/1.1" 200 1234`,
|
|
}
|
|
testTime := time.Date(2024, 1, 2, 3, 4, 5, 0, time.UTC)
|
|
testTimeStr := testTime.Format(LogTimeFormat)
|
|
|
|
for i, test := range tests {
|
|
tests[i] = fmt.Sprintf(test, testTimeStr)
|
|
}
|
|
|
|
for _, test := range tests {
|
|
t.Run(test, func(t *testing.T) {
|
|
actual := ParseLogTime([]byte(test))
|
|
ExpectTrue(t, actual.Equal(testTime))
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestRetentionCommonFormat(t *testing.T) {
|
|
var file MockFile
|
|
logger := NewAccessLoggerWithIO(task.RootTask("test", false), &file, &Config{
|
|
Format: FormatCommon,
|
|
BufferSize: 1024,
|
|
})
|
|
for range 10 {
|
|
logger.Log(req, resp)
|
|
}
|
|
logger.Flush()
|
|
// test.Finish(nil)
|
|
|
|
ExpectEqual(t, logger.Config().Retention, nil)
|
|
ExpectTrue(t, file.Len() > 0)
|
|
ExpectEqual(t, file.LineCount(), 10)
|
|
|
|
t.Run("keep last", func(t *testing.T) {
|
|
logger.Config().Retention = strutils.MustParse[*Retention]("last 5")
|
|
ExpectEqual(t, logger.Config().Retention.Days, 0)
|
|
ExpectEqual(t, logger.Config().Retention.Last, 5)
|
|
ExpectNoError(t, logger.Rotate())
|
|
ExpectEqual(t, file.LineCount(), 5)
|
|
})
|
|
|
|
_ = file.Truncate(0)
|
|
|
|
timeNow := time.Now()
|
|
for i := range 10 {
|
|
logger.Formatter.(*CommonFormatter).GetTimeNow = func() time.Time {
|
|
return timeNow.AddDate(0, 0, -10+i)
|
|
}
|
|
logger.Log(req, resp)
|
|
}
|
|
logger.Flush()
|
|
ExpectEqual(t, file.LineCount(), 10)
|
|
|
|
t.Run("keep days", func(t *testing.T) {
|
|
logger.Config().Retention = strutils.MustParse[*Retention]("3 days")
|
|
ExpectEqual(t, logger.Config().Retention.Days, 3)
|
|
ExpectEqual(t, logger.Config().Retention.Last, 0)
|
|
ExpectNoError(t, logger.Rotate())
|
|
ExpectEqual(t, file.LineCount(), 3)
|
|
rotated := string(file.Content())
|
|
_ = file.Truncate(0)
|
|
for i := range 3 {
|
|
logger.Formatter.(*CommonFormatter).GetTimeNow = func() time.Time {
|
|
return timeNow.AddDate(0, 0, -3+i)
|
|
}
|
|
logger.Log(req, resp)
|
|
}
|
|
ExpectEqual(t, rotated, string(file.Content()))
|
|
})
|
|
}
|