mirror of
https://github.com/yusing/godoxy.git
synced 2025-07-02 21:34:24 +02:00
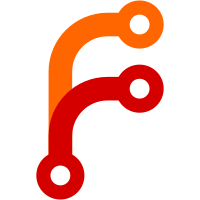
* implement OIDC middleware * auth code cleanup * allow override allowed_user in middleware, fix typos * fix tests and callbackURL * update next release docs * fix OIDC middleware not working with Authentik * feat: add groups support for OIDC claims (#41) Allow users to specify allowed groups in the env and use it to inspect the claims. This performs a logical AND of users and groups (additive). * merge feat/oidc-middleware (#49) * api: enrich provider statistifcs * fix: docker monitor now uses container status * Feat/auto schemas (#48) * use auto generated schemas * go version bump and dependencies upgrade * clarify some error messages --------- Co-authored-by: yusing <yusing@6uo.me> * cleanup some loadbalancer code * api: cleanup websocket code * api: add /v1/health/ws for health bubbles on dashboard * feat: experimental memory logger and logs api for WebUI --------- Co-authored-by: yusing <yusing@6uo.me> --------- Co-authored-by: yusing <yusing@6uo.me> Co-authored-by: Peter Olds <peter@olds.co>
59 lines
1.5 KiB
Go
59 lines
1.5 KiB
Go
package middleware
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/yusing/go-proxy/internal/api/v1/auth"
|
|
E "github.com/yusing/go-proxy/internal/error"
|
|
)
|
|
|
|
type oidcMiddleware struct {
|
|
AllowedUsers []string `json:"allowed_users"`
|
|
AllowedGroups []string `json:"allowed_groups"`
|
|
|
|
auth auth.Provider
|
|
authMux *http.ServeMux
|
|
logoutHandler http.HandlerFunc
|
|
}
|
|
|
|
var OIDC = NewMiddleware[oidcMiddleware]()
|
|
|
|
func (amw *oidcMiddleware) finalize() error {
|
|
if !auth.IsOIDCEnabled() {
|
|
return E.New("OIDC not enabled but ODIC middleware is used")
|
|
}
|
|
authProvider, err := auth.NewOIDCProviderFromEnv()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
authProvider.SetIsMiddleware(true)
|
|
if len(amw.AllowedUsers) > 0 {
|
|
authProvider.SetAllowedUsers(amw.AllowedUsers)
|
|
}
|
|
if len(amw.AllowedGroups) > 0 {
|
|
authProvider.SetAllowedGroups(amw.AllowedGroups)
|
|
}
|
|
|
|
amw.authMux = http.NewServeMux()
|
|
amw.authMux.HandleFunc(auth.OIDCMiddlewareCallbackPath, authProvider.LoginCallbackHandler)
|
|
amw.authMux.HandleFunc(auth.OIDCLogoutPath, func(w http.ResponseWriter, r *http.Request) {
|
|
http.Error(w, "Unauthorized", http.StatusUnauthorized)
|
|
})
|
|
amw.authMux.HandleFunc("/", authProvider.RedirectLoginPage)
|
|
amw.logoutHandler = auth.LogoutCallbackHandler(authProvider)
|
|
amw.auth = authProvider
|
|
return nil
|
|
}
|
|
|
|
func (amw *oidcMiddleware) before(w http.ResponseWriter, r *http.Request) (proceed bool) {
|
|
if err := amw.auth.CheckToken(r); err != nil {
|
|
amw.authMux.ServeHTTP(w, r)
|
|
return false
|
|
}
|
|
if r.URL.Path == auth.OIDCLogoutPath {
|
|
amw.logoutHandler(w, r)
|
|
return false
|
|
}
|
|
return true
|
|
}
|