mirror of
https://github.com/yusing/godoxy.git
synced 2025-07-02 21:34:24 +02:00
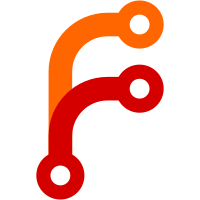
- Incorrect name being shown on dashboard "Proxies page" - Apps being shown when homepage.show is false - Load balanced routes are shown on homepage instead of the load balancer - Route with idlewatcher will now be removed on container destroy - Idlewatcher panic - Performance improvement - Idlewatcher infinitely loading - Reload stucked / not working properly - Streams stuck on shutdown / reload - etc... Added: - support idlewatcher for loadbalanced routes - partial implementation for stream type idlewatcher Issues: - graceful shutdown
55 lines
1.1 KiB
Go
55 lines
1.1 KiB
Go
package loadbalancer
|
|
|
|
import (
|
|
"net/http"
|
|
"time"
|
|
|
|
"github.com/yusing/go-proxy/internal/net/types"
|
|
U "github.com/yusing/go-proxy/internal/utils"
|
|
F "github.com/yusing/go-proxy/internal/utils/functional"
|
|
"github.com/yusing/go-proxy/internal/watcher/health"
|
|
)
|
|
|
|
type (
|
|
Server struct {
|
|
_ U.NoCopy
|
|
|
|
Name string
|
|
URL types.URL
|
|
Weight weightType
|
|
|
|
handler http.Handler
|
|
healthMon health.HealthMonitor
|
|
}
|
|
servers = []*Server
|
|
Pool = F.Map[string, *Server]
|
|
)
|
|
|
|
var newPool = F.NewMap[Pool]
|
|
|
|
func NewServer(name string, url types.URL, weight weightType, handler http.Handler, healthMon health.HealthMonitor) *Server {
|
|
srv := &Server{
|
|
Name: name,
|
|
URL: url,
|
|
Weight: weight,
|
|
handler: handler,
|
|
healthMon: healthMon,
|
|
}
|
|
return srv
|
|
}
|
|
|
|
func (srv *Server) ServeHTTP(rw http.ResponseWriter, r *http.Request) {
|
|
srv.handler.ServeHTTP(rw, r)
|
|
}
|
|
|
|
func (srv *Server) String() string {
|
|
return srv.Name
|
|
}
|
|
|
|
func (srv *Server) Status() health.Status {
|
|
return srv.healthMon.Status()
|
|
}
|
|
|
|
func (srv *Server) Uptime() time.Duration {
|
|
return srv.healthMon.Uptime()
|
|
}
|